This post describes a code that take into consideration temporary anomalies.
Embedded products may be subjected to harsh operating environments and have long durability periods. Therefore, temporary abnormalities that do not result in failures may occur due to the occurrence of noise and other disturbance factors, hardware element degradation, and other factors. There are products that include codes that take such cases into account.
This may seem odd to a newcomer, but it may be a countermeasure to a problem that has occurred in the marketplace, and you can see why.
Example1 : Overflow check for addition and underflow check for subtraction
Some embedded products have rules to include overflow checks for addition and underflow checks for subtraction.
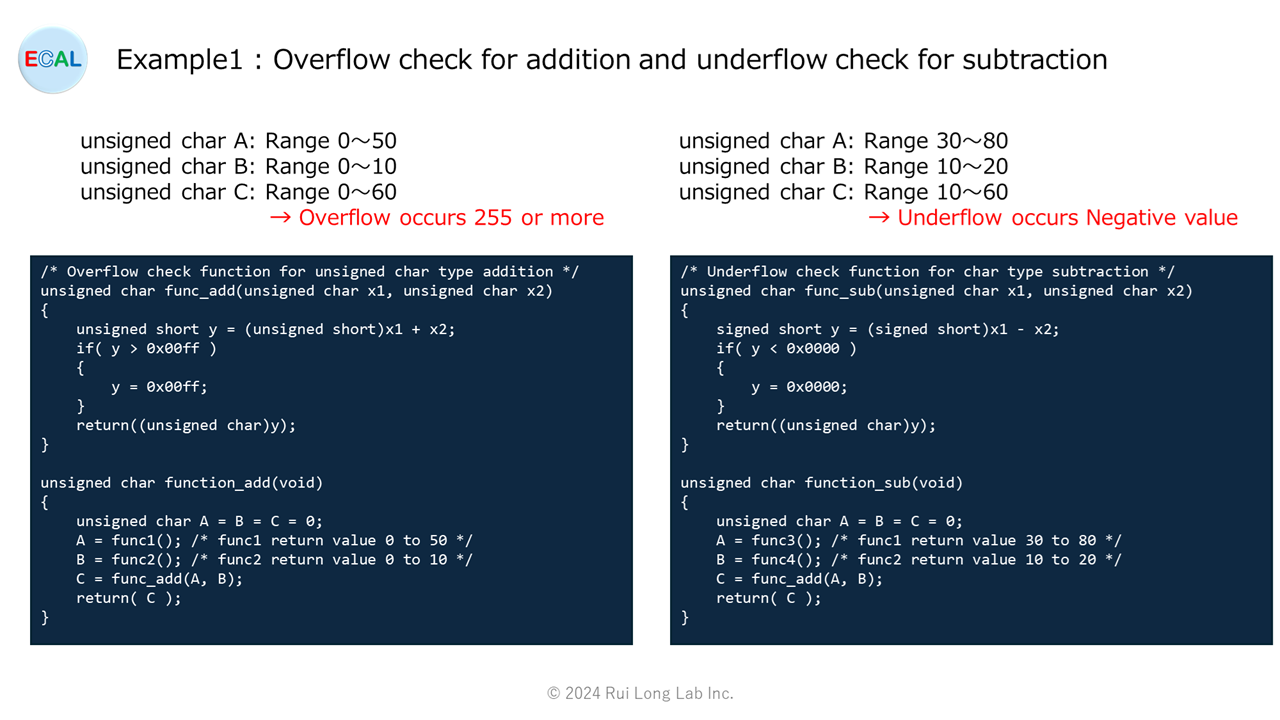
/* Overflow check function for unsigned char type addition */
unsigned char func_add(unsigned char x1, unsigned char x2)
{
unsigned short y = (unsigned short)x1 + x2;
if( y > 0x00ff )
{
y = 0x00ff;
}
return((unsigned char)y);
}
/* Underflow check function for char type subtraction */
unsigned char func_sub(unsigned char x1, unsigned char x2)
{
signed short y = (signed short)x1 - x2;
if( y < 0x0000 )
{
y = 0x0000;
}
return((unsigned char)y);
}
unsigned char function_add(void)
{
unsigned char A = B = C = 0;
A = func1(); /* func1 return value 0 to 50 */
B = func2(); /* func2 return value 0 to 10 */
C = func_add(A, B);
return( C );
}
unsigned char function_sub(void)
{
unsigned char A = B = C = 0;
A = func3(); /* func1 return value 30 to 80 */
B = func4(); /* func2 return value 10 to 20 */
C = func_add(A, B);
return( C );
}
Example 2: AD Conversion Bit Larger AD conversion value
Some embedded products have a rule that unused Bits in microcontroller registers must be read with zero unused Bits.
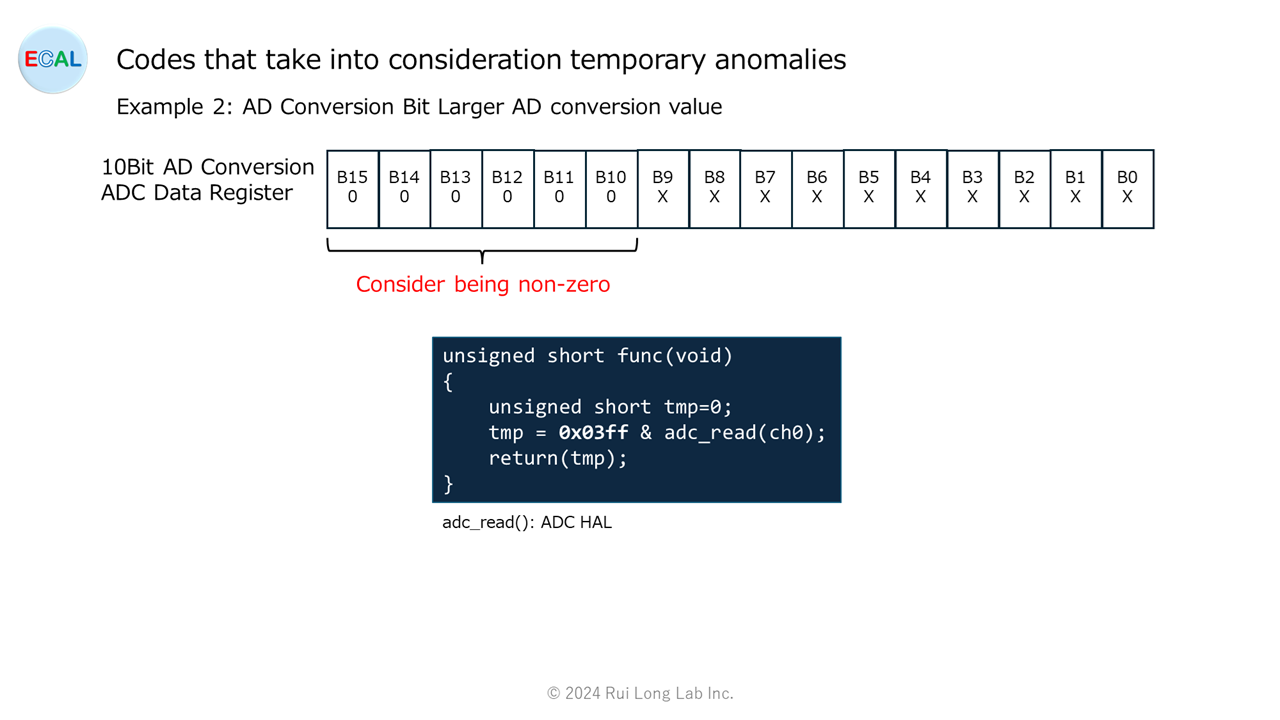
unsigned short func(void)
{
unsigned short tmp=0;
tmp = 0x03ff & adc_read(ch0);
return(tmp);
}