OSS-ECAL stands for Open Source Software for Electronic Components Abstraction Layer. OSS-ECAL is a software layer aimed at streamlining software development for electronic components and improving the availability of electronic components, and is equipped with a software interface tailored to the characteristics of electronic components.
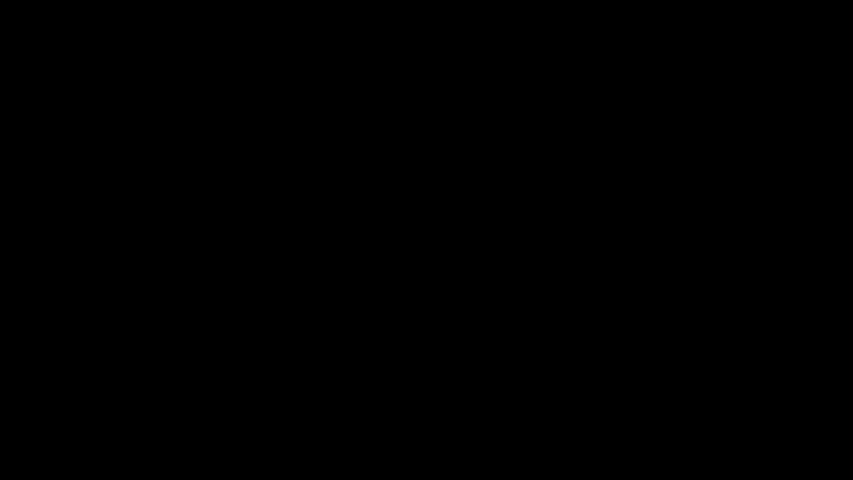
Definition of Terms
Term | Definition |
---|---|
ABC | Temporary name of electronic component |
API | Application interface |
COMMAND | Tentative name of command Name after “eCMD_” declared in etCMD Rename member names to match electronic components |
e (prefix) | Enumerated type member-name |
et (prefix) | Enumerated type tag-name |
etCMD | Enumerated type definition for commands Rename member names to match electronic components |
etSTS | Enumerated type definition for status |
f | Floating-point |
HAL | Hardware Abstraction Layer (AUTOSAR MCAL) |
HALNAME | Tentative name of HAL For HALNAME, please refer to HAL Support. |
i | Integer Fixed-point (prefix) #define immediate |
oABC | API Function Name (Type of command passed as parameter) Rename member names to match electronic components |
oABC_COMMAND | API Function Name Rename member names to match electronic components |
OSS-ECAL | Open Source Software for Electronic Components Abstraction Layer |
READ | Reading from electronic components (receiving, in the case of communication) |
st (prefix) | Struct type tag-name |
stABC_I | Tentative name for argument types of API function inputs |
stABC_O | Tentative name for argument types of API function outputs |
WRITE | Writing to electronic components (send) |
WRITE_READ | 1. Writing to electronic components (send) 2. Read from electronic components (receive) |
API Function
In order to realize standard interfaces for electronic components and interfaces tailored to the characteristics of each electronic component, the API provides the following two types of API functions.
etSTS oABC( etCMD cmd, stABC_I dat, stABC_O* rlt )
This API function type passes commands as parameters.
Function name
Return value
Parameter IN
Parameter OUT
oABC
etSTS
etCMD cmd
stABC_I dat
stABC_O* &rlt
API function name of ABC
OSS-ECAL status code
OSS-ECAL command code
In data of ABC function
Out data of ABC function
Exe : Temperature sensor BD1020HFV manufactured by ROHM. ADC type
etSTS oBD1020HFV( etCMD, float32* );
void function(void)
{
float32 rlt; // Temperature[degrees Celsius]
// Output voltage of BD1020HFV is converted to AD and the result of temperature conversion is
// read into vgPHY (READ command)
etSTS sts = oBD1020HFV( eCMD_START_READ, &rlt );
}
Return value
Parameter IN
Parameter OUT
etSTS
etCMD cmd
float32* &rlt
OSS-ECAL status code
OSS-ECAL command code
Temperature -30 to 100 [°C]
etCMD
eCMD_START
Process :
1. ADC start.
API function : etSTS oBD1020HFV_START( void )
Return value :
eSTS_FIN
eSTS_ERR_HAL_ADC
eSTS_ERR_COMMAND_CODE
eSTS_ERR_OTHERS_RUN
eSTS_ERR_ADC_OBJECT
NOTE : Arduino, Mbeb, ModusToolbox not supported.
eCMD_READ
Process :
1. ADC value read.
2. Converts ADC values to Temperature values.
Voltage value = (AD conversion value × VDD) / (2AD bit )
Temperature value = ((voltage value – voltage offset value) / gain) + Temperature offset value
Min, Max Limit
3. Write Temperature values to rlt.
API function : etSTS oBD1020HFV_READ( float32* &rlt )
Return value :
eSTS_FIN
eSTS_ERR_MIN
eSTS_ERR_MAX
eSTS_ERR_HAL_ADC
eSTS_ERR_COMMAND_CODE
eSTS_ERR_OTHERS_RUN
eSTS_ERR_ADC_OBJECT
NOTE : Arduino, Mbeb, ModusToolbox not supported.
eCMD_START_READ
Process :
1. ADC start.
2. Wait until ADC is completed.
3. ADC value read.
4. Converts ADC values to Temperature values.
Voltage value = (AD conversion value × VDD) / (2AD bit )
Temperature value = ((voltage value – voltage offset value) / gain) + Temperature offset value
Min, Max Limit
5. Write Temperature values to rlt.
API function : etSTS oBD1020HFV_START_READ( float32* &rlt )
Return value :
eSTS_FIN
eSTS_ERR_MIN
eSTS_ERR_MAX
eSTS_ERR_HAL_ADC
eSTS_ERR_COMMAND_CODE
eSTS_ERR_OTHERS_RUN
eSTS_ERR_ADC_OBJECT
NOTE :
etSTS oABC_COMMAND( stABC_I dat, stABC_O* rlt )
This API function type specifies a command by function name. (Command API function)
Function name
Return value
Parameter IN
Parameter OUT
oABC
etSTS
stABC_I dat
stABC_O* rlt
API function name of ABC
OSS-ECAL status code
In data of ABC function
Out data of ABC function
Exe : Temperature sensor BD1020HFV manufactured by ROHM. ADC type
etSTS oBD1020HFV_READ( float32* );
void function(void)
{
float32 rlt; // Temperature[degrees Celsius]
// Output voltage of BD1020HFV is converted to AD and the result of temperature conversion is
// read into vgPHY (READ command)
etSTS sts = oBD1020HFV_READ( &rlt );
}
Return value
Parameter OUT
etSTS
float32* &rlt
OSS-ECAL status code
Temperature -30 to 100 [°C]
etSTS oBD1020HFV_READ( float32* &rlt )
Process :
1. ADC value read.
2. Converts ADC values to Temperature values.
Voltage value = (AD conversion value × VDD) / (2AD bit )
Temperature value = ((voltage value – voltage offset value) / gain) + Temperature offset value
Min, Max Limit
3. Write Temperature values to rlt.
Return value :
eSTS_FIN
eSTS_ERR_MIN
eSTS_ERR_MAX
eSTS_ERR_HAL_ADC
eSTS_ERR_COMMAND_CODE
eSTS_ERR_OTHERS_RUN
eSTS_ERR_ADC_OBJECT
NOTE : Arduino, Mbeb, ModusToolbox not supported.
Files
The file structure of OSS-ECAL is as follows. The structure differs depending on the HAL, so please check the OSS-ECAL page for each electronic component.
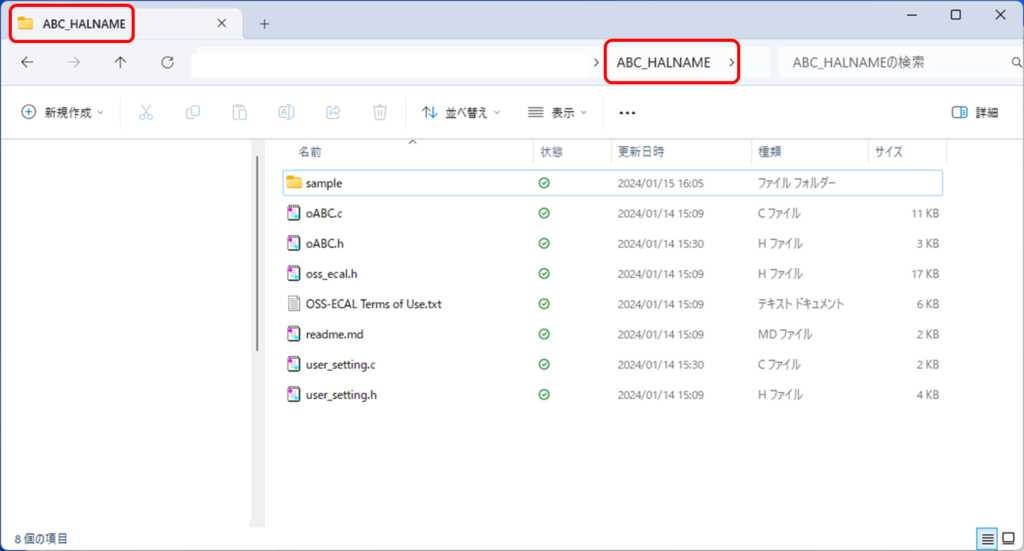
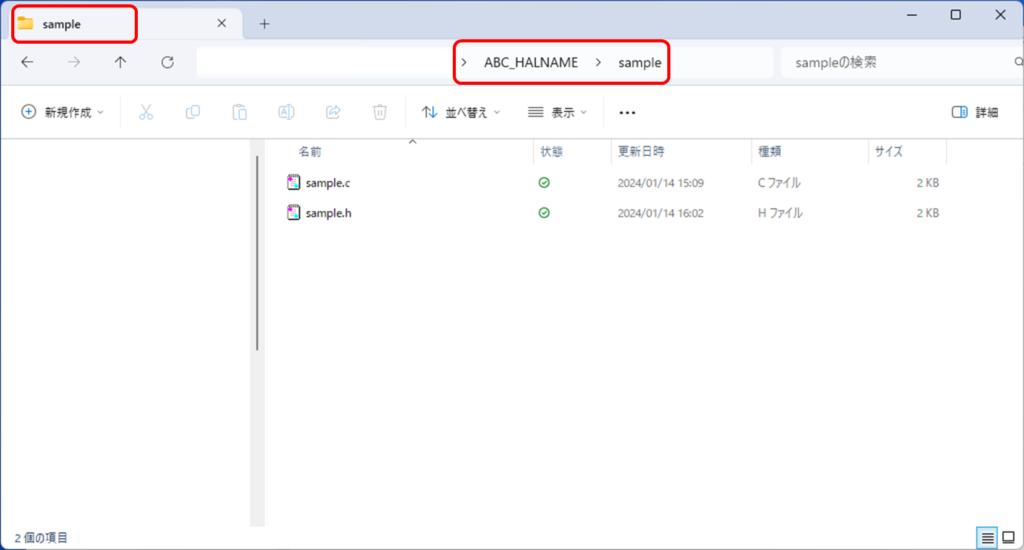
sample.c (.cpp)
sample.h
oABC.c (.cpp)
oABC.h
oss_ecal.h
user_setting.c (.cpp)
user_setting.h
readme.md
OSS-ECAL Terms of Use.txt
Sample Application Program
Sample Application Header
OSS-ECAL Program for ABC
OSS-ECAL Header for ABC
OSS-ECAL Common Header
Const and Table of User settings
Header of User settings
Readme
OSS-ECAL Terms of Use
HAL Support
OSS-ECAL HAL support is as follows (expanding sequentially). Please check each electronic components OSS-ECAL page for HAL support.
MCU/Board manufacturer | HAL | MCU* | Development environment* | HALNAME |
---|---|---|---|---|
Arduino | Arduino 1.8.6 | ATmega2560 ATmega328P | Mega 2560 Rev3 Arduino Pro Mini 3.3V | ARDUINO |
ARM | Mbed 6.17.0 | STM32F401RETx | STM32 Nucleo-64 boards | MBED |
Infineon | ModusToolbox HAL Cat1 2.4.3 | CYBLE-416045-02 | CY8CPROTO-063-BLE PSoC 6 BLE Prototyping Kit | ModusToolbox |
NXP | MCUXpresso SDK iMXRT1051B_1052B ksdk2_0 | CYBLE-416045-02 | IMXRT1050-EVKB | iMXRT1051B1052B |
Renesas | SSP 2.4.0 | R7FS7G27H3A01CFC | S7G2 SK | SSP |
STM | STM32Cube FW_F4 V1.27.1 | STM32F401RETx | STM32 Nucleo-64 boards | STM32F4 |
NXP | AUTOSAR (MCAL) | MPC574XG-100DS | MPC574XG-MB( Motherboard) MPC574XG-100DS(Daughter boards) | MCAL |
* MCU and development environment at the time of development. Even if the same HAL is used, different MCUs and development environments may not work together.
Built-in How to
How to incorporate OSS-ECAL into user programs
How to incorporate multiple OSS-ECALs in a user program (same MCU function)
How to incorporate multiple OSS-ECALs in a user program (different MCU function)
How to incorporate multiple identical electronic components into a user program
How to reduce the impact on user programs by replacing electronic components